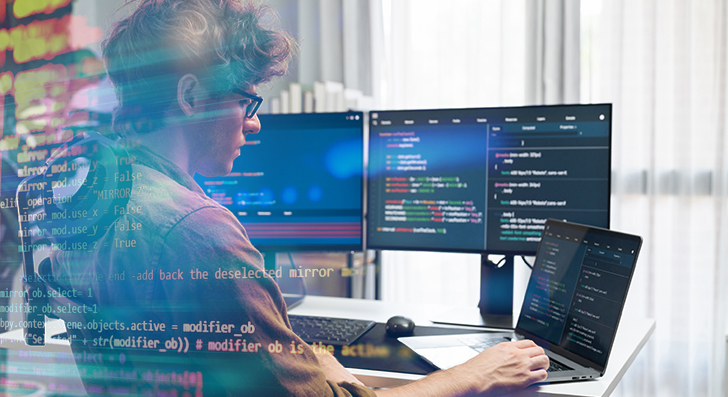
Scalability usually means your application can deal with progress—much more users, additional info, and much more visitors—without breaking. To be a developer, constructing with scalability in mind will save time and anxiety later. Right here’s a transparent and useful manual to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not a little something you bolt on afterwards—it should be part of the plan from the start. Many apps fail whenever they develop fast mainly because the original style and design can’t deal with the additional load. As being a developer, you'll want to Believe early regarding how your program will behave stressed.
Begin by coming up with your architecture to be versatile. Stay clear of monolithic codebases in which anything is tightly connected. As an alternative, use modular style or microservices. These designs crack your application into smaller sized, impartial sections. Each module or support can scale By itself with out impacting The full procedure.
Also, consider your database from day just one. Will it need to handle a million buyers or simply a hundred? Select the appropriate form—relational or NoSQL—based on how your info will increase. Approach for sharding, indexing, and backups early, even if you don’t need to have them still.
A further important point is to prevent hardcoding assumptions. Don’t compose code that only performs underneath latest disorders. Take into consideration what would take place Should your consumer base doubled tomorrow. Would your app crash? Would the database decelerate?
Use structure styles that guidance scaling, like information queues or party-pushed devices. These enable your application take care of far more requests with no acquiring overloaded.
Once you Construct with scalability in mind, you're not just preparing for fulfillment—you might be cutting down foreseeable future head aches. A nicely-planned procedure is less complicated to take care of, adapt, and increase. It’s far better to prepare early than to rebuild afterwards.
Use the appropriate Database
Choosing the ideal databases is actually a essential Portion of building scalable purposes. Not all databases are created the identical, and using the Erroneous one can gradual you down or maybe result in failures as your app grows.
Start by knowledge your knowledge. Is it remarkably structured, like rows within a desk? If Indeed, a relational database like PostgreSQL or MySQL is a great healthy. These are generally strong with associations, transactions, and consistency. Additionally they assistance scaling approaches like go through replicas, indexing, and partitioning to take care of a lot more traffic and details.
Should your details is much more adaptable—like user action logs, product catalogs, or paperwork—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at dealing with large volumes of unstructured or semi-structured knowledge and will scale horizontally much more quickly.
Also, think about your read through and generate patterns. Will you be doing a great deal of reads with fewer writes? Use caching and browse replicas. Are you presently handling a large produce load? Look into databases that will cope with superior create throughput, as well as event-based mostly knowledge storage units like Apache Kafka (for temporary info streams).
It’s also sensible to Assume in advance. You might not require Superior scaling characteristics now, but choosing a database that supports them indicates you gained’t need to have to change later on.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your details depending on your access designs. And constantly keep an eye on databases effectiveness while you increase.
Briefly, the appropriate databases will depend on your application’s construction, velocity desires, And just how you be expecting it to improve. Acquire time to choose correctly—it’ll preserve plenty of problems later.
Optimize Code and Queries
Quick code is key to scalability. As your application grows, each individual smaller delay adds up. Poorly penned code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s vital that you Create effective logic from the start.
Get started by producing clear, easy code. Avoid repeating logic and take away everything pointless. Don’t pick the most advanced Remedy if an easy 1 is effective. Maintain your functions shorter, targeted, and easy to check. Use profiling resources to uncover bottlenecks—spots exactly where your code usually takes much too prolonged to run or works by using excessive memory.
Subsequent, evaluate your database queries. These often sluggish things down in excess of the code itself. Ensure that Just about every query only asks for the info you actually need to have. Avoid Decide on *, which fetches every thing, and as a substitute choose distinct fields. Use indexes to hurry up lookups. And stay away from accomplishing a lot of joins, especially across substantial tables.
In the event you observe the same info staying requested over and over, use caching. Retail outlet the results temporarily employing resources like Redis or Memcached therefore you don’t have to repeat pricey functions.
Also, batch your databases functions when you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and makes your app a lot more successful.
Remember to check with huge datasets. Code and queries that get the job done great with 100 records may crash whenever they have to manage one million.
To put it briefly, scalable applications are speedy applications. Keep your code restricted, your queries lean, and use caching when necessary. These methods enable your software keep sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of additional buyers plus more site visitors. If every little thing goes by way of one particular server, it is going to speedily become a bottleneck. That’s in which load balancing and caching are available in. These two tools help keep your application rapid, steady, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. As an alternative to one particular server carrying out each of the function, the load balancer routes users to distinctive servers dependant on availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out visitors to the Other folks. Resources like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to create.
Caching is about storing information quickly so it could be reused rapidly. When users ask for the identical information yet again—like an item website page or perhaps a profile—you don’t really need to fetch it through the database when. It is possible to serve it with the cache.
There are two popular forms of caching:
1. Server-aspect caching (like Redis or Memcached) stores facts in memory for rapidly access.
two. Client-aspect caching (like browser caching or CDN caching) stores static documents near the consumer.
Caching cuts down database load, increases speed, and would make your app far more efficient.
Use caching for things which don’t alter normally. And often be certain your cache is up to date when details does modify.
To put it briefly, load balancing and caching are straightforward but impressive resources. Jointly, they assist your app take care of more consumers, stay quickly, and Get well from problems. If you intend to improve, you need the two.
Use Cloud and Container Instruments
To build scalable programs, you may need applications that permit your app expand simply. That’s where by cloud platforms and containers come in. They provide you overall flexibility, cut down setup time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and services as you'll need them. You don’t must get components or guess long run ability. When targeted visitors increases, you'll be able to incorporate far more methods with just a couple clicks or mechanically working with vehicle-scaling. When website traffic drops, you could scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and protection instruments. It is possible to center on making your app in place of taking care of infrastructure.
Containers are A different essential Device. A container packages your application and all the things it ought to operate—code, libraries, options—into 1 device. This causes it to be effortless to move your application involving environments, out of your laptop into the cloud, with out surprises. Docker is the preferred Resource for this.
Whenever your app works by using a number of containers, resources like Kubernetes help you regulate them. Kubernetes handles deployment, scaling, and Restoration. If 1 section of your respective app crashes, it restarts it quickly.
Containers also help it become simple to different areas of your app into services. You may update or scale elements independently, which is perfect for overall performance and trustworthiness.
In brief, applying cloud and container equipment means you may scale quick, deploy quickly, and Recuperate immediately when difficulties materialize. If you'd like your application to develop devoid of boundaries, start employing these applications early. They preserve time, lower danger, and make it easier to stay focused on making, not fixing.
Check All the things
In the event you don’t keep an eye on your software, you won’t know when items go Erroneous. Checking assists you see how your application is carrying out, place difficulties early, and make better decisions as your app grows. It’s a essential Element of building scalable techniques.
Start off by monitoring essential metrics like CPU usage, memory, disk Area, and response time. These let you know how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just watch your servers—keep track of your app also. Keep watch over just how long it requires for people to load web pages, how frequently glitches materialize, and where by they manifest. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for vital problems. For example, if your reaction time goes higher than a Restrict or maybe a service goes down, you'll want to get notified straight away. This allows you deal with difficulties rapidly, typically just before consumers even discover.
Checking is usually handy if you make adjustments. In the event you deploy a new aspect and find out a spike in mistakes or slowdowns, you are able to roll it again ahead of it triggers real destruction.
As your application grows, targeted traffic and info increase. Devoid of monitoring, you’ll miss indications of difficulty right until it’s way too late. But with the correct applications in position, you stay on top of things.
In brief, checking aids you keep the app responsible and scalable. It’s not almost recognizing failures—it’s about comprehension your method and making certain it works very well, even under pressure.
Closing Ideas
Scalability isn’t only for huge providers. Even modest applications want a solid foundation. By coming up with cautiously, optimizing correctly, and utilizing the proper applications, you'll be able to Establish Gustavo Woltmann news apps that increase effortlessly without having breaking stressed. Begin modest, Imagine huge, and Make smart.